個人練習javascriptブロック崩し解説なし
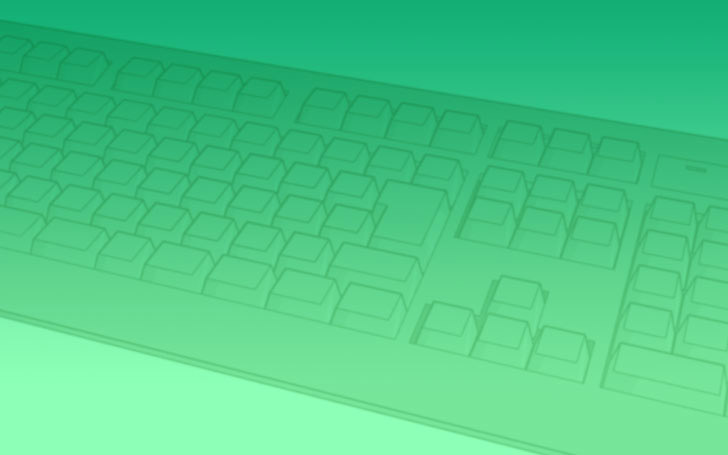
関連タイピング
-
日本語訳なし、個人練習用に作った英語長文です
プレイ回数4108英語長文673打 -
個人練習用で作りました。解説なしです。
プレイ回数1262英語長文1140打 -
解説なし、個人練習用につくりました。
プレイ回数684英語長文1054打 -
jQuery基本例文集です。解説なしです。
プレイ回数940英語長文1554打 -
ワードプレスでよく使う分岐文です。
プレイ回数698長文記号1049打 -
解説付きでbox-shadowの基本をまとめています。
プレイ回数290長文315打 -
h2やh3のデザインのcssです。解説なしです。
プレイ回数325英語長文980打 -
個人練習用:サイトパーツ、アコーディオン
プレイ回数149英語長文1379打
問題文
(function setup() {)
function setup() {
(createCanvas(400, 400);)
createCanvas(400, 400);
(for (let i = 0; i < 12; i++) {)
for (let i = 0; i < 12; i++) {
(let p = new Vec2(90*(i%4)+50, 50*floor(i/4)+50);)
let p = new Vec2(90*(i%4)+50, 50*floor(i/4)+50);
(blocks.push(new Block(p, 20)); } })
blocks.push(new Block(p, 20)); } }
(class Vec2 {)
class Vec2 {
(constructor(_x, _y) {)
constructor(_x, _y) {
(this.x = _x;)
this.x = _x;
(this.y = _y; })
this.y = _y; }
(add(b) {)
add(b) {
(let a = this;)
let a = this;
(return new Vec2(a.x + b.x, a.y + b.y); })
return new Vec2(a.x + b.x, a.y + b.y); }
(mul(s) { let a = this;)
mul(s) { let a = this;
(return new Vec2(s * a.x, s * a.y); })
return new Vec2(s * a.x, s * a.y); }
(mag() {)
mag() {
(let a = this;)
let a = this;
(return sqrt(a.x ** 2 + a.y ** 2); })
return sqrt(a.x ** 2 + a.y ** 2); }
(sub(b) { let a = this;)
sub(b) { let a = this;
(return new Vec2(a.x - b.x, a.y - b.y); })
return new Vec2(a.x - b.x, a.y - b.y); }
(norm() {)
norm() {
(let a = this;)
let a = this;
(return a.mul(1 / a.mag()); })
return a.mul(1 / a.mag()); }
(dot(b) {)
dot(b) {
(let a = this;)
let a = this;
(return a.x * b.x + a.y * b.y; })
return a.x * b.x + a.y * b.y; }
(reflect(w) {)
reflect(w) {
(let v = this;)
let v = this;
(let cosTheta = v.mul(-1))
let cosTheta = v.mul(-1)
(.dot(w) / (v.mul(-1).mag() * w.mag());)
.dot(w) / (v.mul(-1).mag() * w.mag());
(let n = w.norm().mul(v.mag() * cosTheta);)
let n = w.norm().mul(v.mag() * cosTheta);
(let r = v.add(n.mul(2));)
let r = v.add(n.mul(2));
(return r; } })
return r; } }
(class Ball {)
class Ball {
(constructor(_p, _v, _r) {)
constructor(_p, _v, _r) {
(this.p = _p;)
this.p = _p;
(this.v = _v;)
this.v = _v;
(this.r = _r; } })
this.r = _r; } }
(class Block {)
class Block {
(constructor(_p, _r) {)
constructor(_p, _r) {
(this.p = _p;)
this.p = _p;
(this.r = _r; } })
this.r = _r; } }
(class Paddle {)
class Paddle {
(constructor(_p, _r) {)
constructor(_p, _r) {
(this.p = _p;)
this.p = _p;
(this.r = _r; } })
this.r = _r; } }
(let ball = new Ball()
let ball = new Ball(
(new Vec2(200, 300),)
new Vec2(200, 300),
(new Vec2(240, -60),)
new Vec2(240, -60),
(15 );)
15 );
(let blocks = [];)
let blocks = [];
(let paddle = new Paddle(new Vec2(200, 320), 30);)
let paddle = new Paddle(new Vec2(200, 320), 30);
(function draw() {)
function draw() {
(ball.p = ball.p.add(ball.v.mul(1 / 60));)
ball.p = ball.p.add(ball.v.mul(1 / 60));
(if ((ball.p.x < 15) || (ball.p.x > 385)) {)
if ((ball.p.x < 15) || (ball.p.x > 385)) {
(ball.v.x = -ball.v.x; })
ball.v.x = -ball.v.x; }
(if ((ball.p.y < 15) || (ball.p.y > 385)) {)
if ((ball.p.y < 15) || (ball.p.y > 385)) {
(ball.v.y = -ball.v.y; })
ball.v.y = -ball.v.y; }
(for (let block of blocks) {)
for (let block of blocks) {
(let d = block.p.sub(ball.p).mag();)
let d = block.p.sub(ball.p).mag();
(if (d < (ball.r + block.r)) {)
if (d < (ball.r + block.r)) {
(let w = ball.p.sub(block.p);)
let w = ball.p.sub(block.p);
(let r = ball.v.reflect(w);)
let r = ball.v.reflect(w);
(ball.v = r;)
ball.v = r;
(blocks.splice(blocks.indexOf(block), 1);)
blocks.splice(blocks.indexOf(block), 1);
(} })
} }
(paddle.p.x = mouse-X;)
paddle.p.x = mouse-X;
(let d = paddle.p.sub(ball.p).mag();)
let d = paddle.p.sub(ball.p).mag();
(if (d < (ball.r + paddle.r)) {)
if (d < (ball.r + paddle.r)) {
(let w = ball.p.sub(paddle.p);)
let w = ball.p.sub(paddle.p);
(let r = ball.v.reflect(w);)
let r = ball.v.reflect(w);
(ball.v = r;)
ball.v = r;
(ball.p = paddle.p.add(w.norm())
ball.p = paddle.p.add(w.norm()
(.mul(ball.r + paddle.r)); })
.mul(ball.r + paddle.r)); }
(background(220);)
background(220);
(circle(ball.p.x, ball.p.y, 2*ball.r);)
circle(ball.p.x, ball.p.y, 2*ball.r);
(for (let b of blocks) {)
for (let b of blocks) {
(circle(b.p.x, b.p.y, 2*b.r); })
circle(b.p.x, b.p.y, 2*b.r); }
(circle(paddle.p.x,)
circle(paddle.p.x,
(paddle.p.y, 2*paddle.r); })
paddle.p.y, 2*paddle.r); }