プログラミングPythonタイピング
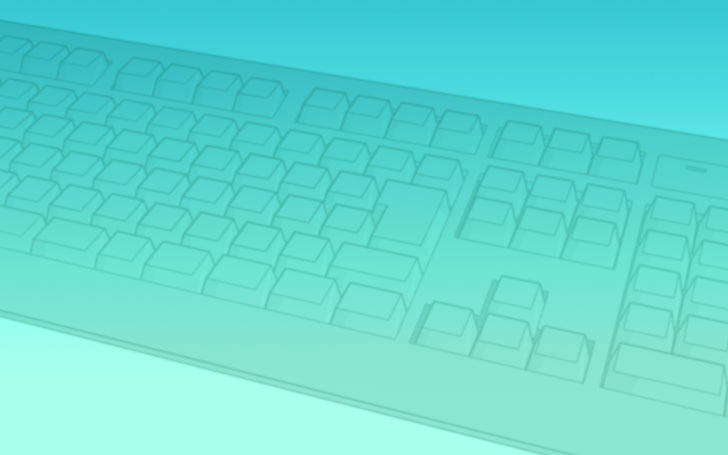
順位 | 名前 | スコア | 称号 | 打鍵/秒 | 正誤率 | 時間(秒) | 打鍵数 | ミス | 問題 | 日付 |
---|---|---|---|---|---|---|---|---|---|---|
1 | ku | 5450 | スーパーエンジニア | 5.5 | 99.0% | 60.0 | 330 | 3 | 13 | 2025/01/06 |
2 | ku | 5216 | エキスパート | 5.2 | 99.0% | 60.0 | 316 | 3 | 11 | 2025/02/01 |
3 | Nao | 4983 | 上級プログラマ | 5.1 | 97.4% | 60.0 | 307 | 8 | 14 | 2025/02/02 |
4 | nakau | 4300 | 情報科学研究者 | 4.5 | 95.7% | 60.0 | 270 | 12 | 13 | 2025/02/23 |
5 | a | 4016 | 情報科学大学院博士後期 | 4.1 | 98.0% | 60.0 | 246 | 5 | 9 | 2025/02/22 |
関連タイピング
-
Mrs.GREEN APPLEの青と夏です!
プレイ回数8万歌詞1030打 -
タイピング練習に関する長文です
プレイ回数9.3万長文1159打 -
10秒以内に打てたら指が神ってる!(?)
プレイ回数6782154打 -
Mrs.グリンアップルのライラックのサビ曲タイピングです
プレイ回数8446歌詞かな173打 -
「映画ドラえもんのび太の地球交響曲」
プレイ回数1588歌詞754打 -
恋愛の小説です
プレイ回数656長文1015打 -
リクエスト
プレイ回数82長文1000打 -
出来るだけ明るめの英文ニュースのタイピングです。
プレイ回数133英語長文458打
問題文
(import os)
import os
(import sys)
import sys
(from datetime import datetime)
from datetime import datetime
(class SampleClass:)
class SampleClass:
(def __init__(self, value):)
def __init__(self, value):
(self.value = value)
self.value = value
(def get_value(self):)
def get_value(self):
(return self.value)
return self.value
(if __name__ == "__main__":)
if __name__ == "__main__":
(sample = SampleClass(10))
sample = SampleClass(10)
(print(sample.get_value()))
print(sample.get_value())
(def add_numbers(a, b):)
def add_numbers(a, b):
(return a + b)
return a + b
(def subtract_numbers(a, b):)
def subtract_numbers(a, b):
(return a - b)
return a - b
(def multiply_numbers(a, b):)
def multiply_numbers(a, b):
(return a * b)
return a * b
(def divide_numbers(a, b):)
def divide_numbers(a, b):
(if b != 0:)
if b != 0:
(return a / b)
return a / b
(return None)
return None
(try:)
try:
(result = divide_numbers(10, 0))
result = divide_numbers(10, 0)
(if result is None:)
if result is None:
(raise ValueError("Cannot divide by zero"))
raise ValueError("Cannot divide by zero")
(except ValueError as e:)
except ValueError as e:
(print(e))
print(e)
(for i in range(5):)
for i in range(5):
(print(f"Iteration: {i}"))
print(f"Iteration: {i}")
(with open("file.txt", "w") as f:)
with open("file.txt", "w") as f:
(f.write("Hello, world!"))
f.write("Hello, world!")
(data = [1, 2, 3, 4, 5])
data = [1, 2, 3, 4, 5]
(squared = [x ** 2 for x in data])
squared = [x ** 2 for x in data]
(filtered = [x for x in data if x % 2 == 0])
filtered = [x for x in data if x % 2 == 0]
(def factorial(n):)
def factorial(n):
(if n == 0:)
if n == 0:
(return 1)
return 1
(return n * factorial(n - 1))
return n * factorial(n - 1)
(def greet(name="Guest"):)
def greet(name="Guest"):
(return f"Hello, {name}!")
return f"Hello, {name}!"
(d = {"name": "Alice", "age": 30})
d = {"name": "Alice", "age": 30}
(if "name" in d:)
if "name" in d:
(print(d["name"]))
print(d["name"])
(name = input("Enter your name: "))
name = input("Enter your name: ")
(print(greet(name)))
print(greet(name))
(import json)
import json
(data = {"key": "value"})
data = {"key": "value"}
(json_str = json.dumps(data))
json_str = json.dumps(data)
(parsed_data = json.loads(json_str))
parsed_data = json.loads(json_str)
(current_time = datetime.now())
current_time = datetime.now()
(print(current_time.strftime("%Y-%m-%d %H:%M:%S")))
print(current_time.strftime("%Y-%m-%d %H:%M:%S"))
(while True:)
while True:
(command = input("Enter command: "))
command = input("Enter command: ")
(if command == "exit":)
if command == "exit":
(break)
break
(numbers = [1, 2, 3, 4, 5])
numbers = [1, 2, 3, 4, 5]
(total = sum(numbers))
total = sum(numbers)
(def is_even(number):)
def is_even(number):
(return number % 2 == 0)
return number % 2 == 0
(if is_even(4):)
if is_even(4):
(print("Number is even"))
print("Number is even")
(set_a = {1, 2, 3})
set_a = {1, 2, 3}
(set_b = {3, 4, 5})
set_b = {3, 4, 5}
(intersection = set_a & set_b)
intersection = set_a & set_b
(union = set_a | set_b)
union = set_a | set_b
(def make_greeting(time_of_day, name):)
def make_greeting(time_of_day, name):
(return f"Good {time_of_day}, {name}!")
return f"Good {time_of_day}, {name}!"
(import random)
import random
(random_number = random.randint(1, 100))
random_number = random.randint(1, 100)
(print(f"Random number: {random_number}"))
print(f"Random number: {random_number}")
(def safe_divide(a, b):)
def safe_divide(a, b):
(try:)
try:
(return a / b)
return a / b
(except ZeroDivisionError:)
except ZeroDivisionError:
(return "Error: Division by zero")
return "Error: Division by zero"
(list_a = [1, 2, 3])
list_a = [1, 2, 3]
(list_b = [4, 5, 6])
list_b = [4, 5, 6]
(combined = list_a + list_b)
combined = list_a + list_b
(config = {"debug": True, "port": 8080})
config = {"debug": True, "port": 8080}
(if config["debug"]:)
if config["debug"]:
(print("Debug mode is on"))
print("Debug mode is on")
(def reverse_string(s):)
def reverse_string(s):
(return s[::-1])
return s[::-1]
(reversed_str = reverse_string("Hello"))
reversed_str = reverse_string("Hello")
(print(reversed_str))
print(reversed_str)
(def find_max(numbers):)
def find_max(numbers):
(return max(numbers))
return max(numbers)
(max_value = find_max([1, 2, 3, 4, 5]))
max_value = find_max([1, 2, 3, 4, 5])
(print(f"Max value: {max_value}"))
print(f"Max value: {max_value}")
(def count_vowels(s):)
def count_vowels(s):
(return sum(1 for char in s if char.lower() in "aeiou"))
return sum(1 for char in s if char.lower() in "aeiou")
(vowel_count = count_vowels("OpenAI"))
vowel_count = count_vowels("OpenAI")
(print(f"Vowel count: {vowel_count}"))
print(f"Vowel count: {vowel_count}")
(def is_palindrome(s):)
def is_palindrome(s):
(return s == s[::-1])
return s == s[::-1]
(print(is_palindrome("racecar")))
print(is_palindrome("racecar"))
(def fibonacci(n):)
def fibonacci(n):
(if n <= 1:)
if n <= 1:
(return n)
return n
(return fibonacci(n - 1) + fibonacci(n - 2))
return fibonacci(n - 1) + fibonacci(n - 2)